Alright, so a lot of people tend to make separate ores for the separate dimensions. This isn't really necessary. You can generate the exact same ores in different dimensions. "But what about textures?" you may ask, to which I will reply with arrays and if statements.
Now, enough blabbering, here is some example code I just wrote for the next Flaxbeard's Steam Power update
As you can see, there are only 3 metadata blocks: Copper, Zinc, and Poor Zinc.
As you can also see, there is an array of 7, each icon in the array is a new texture. I still don't know how to dynamically /create/ textures. Then, it just checks what dimension it's at with Minecraft.getMinecraft().theWorld.provider.dimensionId. It even works with the blocks in your inv! As soon as you exit that dimension, it'll change the texture.
Just thought yall should know about this
Edit:
Because it was requested, pics:
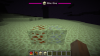
Now, enough blabbering, here is some example code I just wrote for the next Flaxbeard's Steam Power update
Code:
public class BlockSteamcraftOre extends Block {
public IIcon[] icon = new IIcon[7];
public BlockSteamcraftOre() {
super(Material.rock);
setResistance(5.0F);
setHardness(1.5F);
setStepSound(Block.soundTypeStone);
}
@Override
@SideOnly(Side.CLIENT)
public void registerBlockIcons(IIconRegister ir) {
this.icon[0] = ir.registerIcon("steamcraft:oreCopper");
this.icon[1] = ir.registerIcon("steamcraft:oreZinc");
this.icon[2] = ir.registerIcon("steamcraft:poorOreZinc");
this.icon[3] = ir.registerIcon("steamcraft:copper_nether");
this.icon[4] = ir.registerIcon("steamcraft:zinc_nether");
this.icon[5] = ir.registerIcon("steamcraft:copper_end");
this.icon[6] = ir.registerIcon("steamcraft:zinc_end");
}
@SideOnly(Side.CLIENT)
@Override
public IIcon getIcon(int par1, int par2) {
int dimensionID = Minecraft.getMinecraft().theWorld.provider.dimensionId;
if (dimensionID == 0) {
if (par2 == 0) {
return this.icon[0];
}
if (par2 == 1) {
return this.icon[1];
}
if (par2 == 2) {
return this.icon[2];
}
}
if (dimensionID == -1){
if (par2 == 0){
return this.icon[3];
}
if (par2 == 1){
return this.icon[4];
}
}
if (dimensionID == 1){
if (par2 == 0){
return this.icon[5];
}
if (par2 == 1){
return this.icon[6];
}
}
return this.icon[0];
}
@SideOnly(Side.CLIENT)
public void getSubBlocks(Item par1, CreativeTabs par2CreativeTabs, List par3List) {
par3List.add(new ItemStack(par1, 1, 0));
par3List.add(new ItemStack(par1, 1, 1));
if (Loader.isModLoaded("Railcraft") && Config.genPoorOre) {
par3List.add(new ItemStack(par1, 1, 2));
}
}
As you can see, there are only 3 metadata blocks: Copper, Zinc, and Poor Zinc.
As you can also see, there is an array of 7, each icon in the array is a new texture. I still don't know how to dynamically /create/ textures. Then, it just checks what dimension it's at with Minecraft.getMinecraft().theWorld.provider.dimensionId. It even works with the blocks in your inv! As soon as you exit that dimension, it'll change the texture.
Just thought yall should know about this

Edit:
Because it was requested, pics:
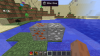
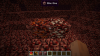
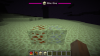
Last edited: